AS3 garbage collection monitor
I needed to watch objects to know if they were properly garbage collected or not, so I've added a GC Monitor in the SomaCore Debugger. It is pretty easy to use and I think very useful if you start to care about the memory that the Flash Player is using and global performance.
Demo Flash (garbage collection monitor)
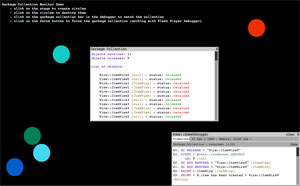
The concept to use it is to "register" an object, such as a Sprite or anything, with a name.
The first step is to create the debugger. If you're using the framework SomaCore, you'll find some demo by clicking here. And if you want to use the debugger without using SomaCore, here is a snippet to create the application and debugger:
package {
import flash.display.Sprite;
import com.soma.core.Soma;
import com.soma.core.interfaces.ISoma;
import com.soma.debugger.SomaDebugger;
import com.soma.debugger.vo.SomaDebuggerVO;
import com.soma.debugger.events.SomaDebuggerEvent;
public class Main extends Sprite {
function Main() {
// create soma application
var app:ISoma = new Soma(stage);
// create debugger options
var vo:SomaDebuggerVO = new SomaDebuggerVO(app, SomaDebugger.NAME_DEFAULT, [], true, false);
// create debugger
var debugger:SomaDebugger = app.createPlugin(SomaDebugger, vo) as SomaDebugger;
// use debugger
debug("Hello Debugger");
debug(this);
debug(app);
}
private function debug(obj:Object):void {
// use app.dispatchEvent() from a class that is not in the display list
dispatchEvent(new SomaDebuggerEvent(SomaDebuggerEvent.PRINT, obj));
// events available:
// SomaDebuggerEvent.SHOW_DEBUGGER;
// SomaDebuggerEvent.CLEAR;
// SomaDebuggerEvent.PRINT;
// SomaDebuggerEvent.HIDE_DEBUGGER;
// SomaDebuggerEvent.MOVE_TO_TOP;
}
}
}
Register an object to watch it:
dispatchEvent(new SomaDebuggerGCEvent(SomaDebuggerGCEvent.ADD\_WATCHER, "my sprite", mySprite));
You can dispatch this event from anywhere in an DisplayObject in the display list (Sprite, MovieClip, etc), from the document class, the stage or the SomaCore instance.
In the debugger window, there is a "garbage collection bar" where you can click to see the registered objects, they can be either "retained" or "released". A released object means it has been garbage collected and doesn't exist anymore. The memory used by this object will be free to use.
A "force" button will trigger the garbage collection, it will call System.gc() twice with a small interval. This should only works in a Flash Player Debugger version, but useful for debugging.
Here is a list of events you can use for the garbage collection monitor:
SomaDebuggerGCEvent.REMOVE_ALL_WATCHERS
SomaDebuggerGCEvent.REMOVE_WATCHER // pass the name of he object as parameter
SomaDebuggerGCEvent.ADD_WATCHER // pass the name of the objects and the object itself as parameter
SomaDebuggerGCEvent.FORCE_GC
Note: by default SomaDebugger is now using a weak reference to the objects that you want to inspect. You can turn off this option to inspect everything in the debugger, but the objects will never be released, making the garbage collection monitor useless.
Here is how to force the inspection of any objects:
SomaDebugger.WEAK_REFERENCE = false;
Any feedback appreciated!
Romu